요르딩딩
[Personal_Project] batch, scheduler 만들기 (6) 본문
이번시간에는 배치를 적용해보는 시간을 가져보겠습니다.
Spring 일괄 처리(batch processing) 배치를 만드는 여러가지의 기능이 있다.
방법1) quartz 스케줄링 객체 사용
방법2) <task:scheduler> 설정 사용
방법3) @Scheduled 어노테이션 사용
방법1) quartz 스케줄링 객체 사용
1. quartz를 사용하기 위해서 <dependency>에 추가해줍니다.
[pom.xml]
<!-- scheduler -->
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz</artifactId>
<version>2.2.0</version>
</dependency>
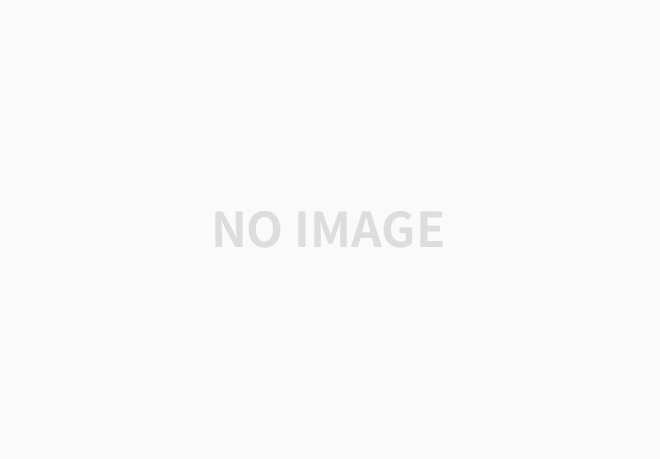
2. 스케줄링 관련 설정을 작성해줍니다.
[context-scheduler.xml]
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd">
<!-- 컴포넌트 스캔 -->
<context:component-scan base-package="com">
</context:component-scan>
<!-- 1. quartz 스케줄링 객체 사용 -->
<!-- 테스트용 잡 -->
<bean id="testJob" class="org.springframework.scheduling.quartz.MethodInvokingJobDetailFactoryBean">
<!-- 서비스 구현 객체의 빈 이름을 인자로 줍니다. (ServiceImpl(@Service("")) -->
<property name="targetObject" ref="batchService" />
<!-- 서비스 객체에서 주기적으로 실행될 메소드른 지정합니다. (ServiceImpl(void method()))-->
<property name="targetMethod" value="batchMethod" />
<!-- 동시 실행을 방지합니다. -->
<property name="concurrent" value="false" />
</bean>
<!-- 테스트용 트리거 -->
<bean id="testJobTrigger" class="org.springframework.scheduling.quartz.CronTriggerFactoryBean">
<property name="jobDetail" ref="testJob" />
<!-- CronTrigger를 사용하여 2분 간격으로 실행되도록 지정했습니다. -->
<property name="cronExpression" value="0 5 21 * * ?" />
</bean>
<!-- 테스트용 스케줄러 -->
<bean id="testJobScheduler" class="org.springframework.scheduling.quartz.SchedulerFactoryBean">
<property name="triggers">
<!-- 앞에서 설정한 트리거를 등록합니다. 필요하면 여러개 만들어서 등록하면 됩니다. -->
<list>
<ref bean="testJobTrigger" />
</list>
</property>
</bean>
</beans>
(1) <컴포넌트 스캔>을 추가해야 등록된 빈을 읽을 수 있습니다.
(2-1) <property name="targetObject" ref="batchService" /> 에 @Service빈으로 등록한 이름을 작성하여줍니다.
(2-2) <property name="targetMethod" value="batchMathod" />에 동작시킬 메소드 이름을 작성하여줍니다.
(3) 트리거의 <property name="cronExpression" value="0 5 21 * * ?" />부분을 통해 스케줄될 시간형식을 작성해줍니다.
(4) 마지막으로 트리거를 동작시켜줍니다.
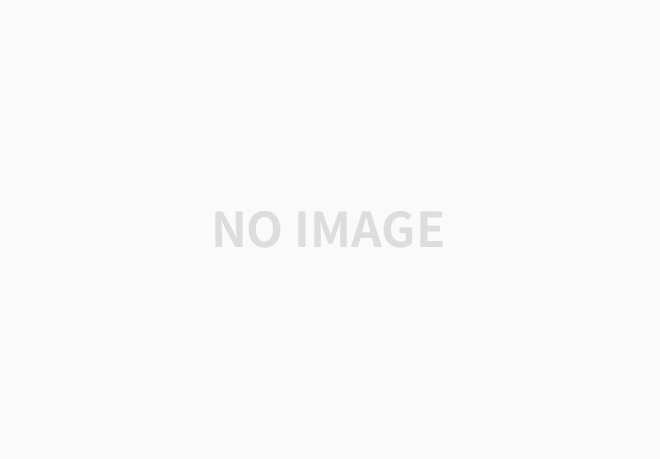
3. service 인터페이스 작성
package com.service;
public interface batchService {
public void batchMethod ();
}
스케줄될 메소드는 void형식이어야 정상적으로 빌드됩니다.
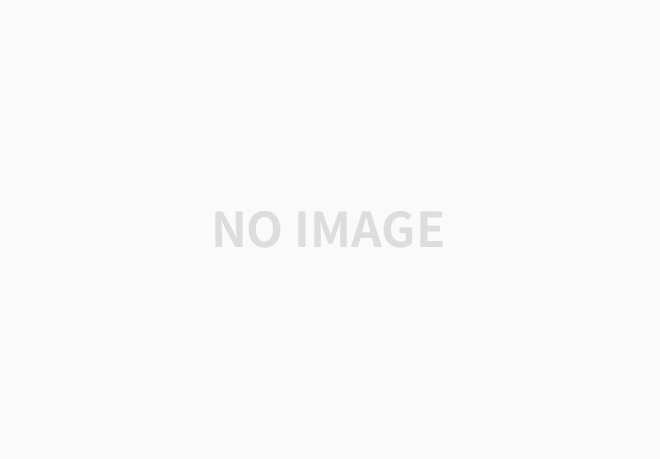
4. service 구현
package com.service.serviceImpl;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
@Service("batchService")
public class batchServiceImpl {
//배치스케줄러 test용
public void batchMethod() {
System.out.println("배치스케줄러가 정상적으로 동작합니다.");
}
}
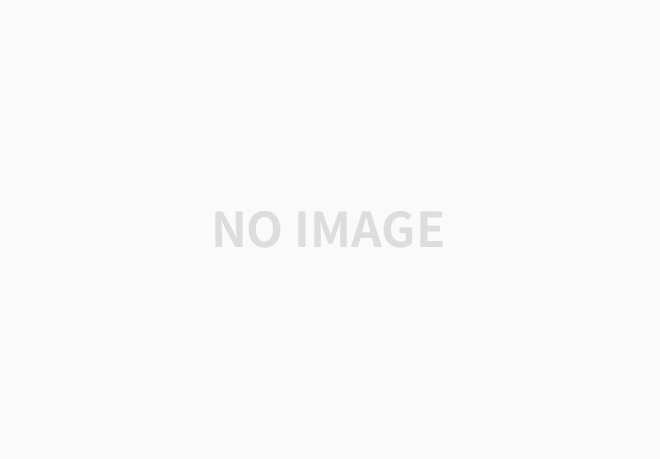
방법2) <task:scheduler> 설정 사용
1. 스케줄링 관련 설정을 작성해줍니다.
[context-scheduler.xml]
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-4.0.xsd">
<!--컴포넌트 스켄을 해야만 @컴포넌트 어노테이션을 인식 할 수 있다. -->
<context:component-scan base-package="com" />
<!-- task 를 @ 애너테이션 기반으로 자동 검색 -->
<task:annotation-driven />
<task:scheduler id=“testJob” pool-size="2"/>
<task:scheduled-tasks scheduler="testJob" >
<task:scheduled ref="batchService" method= "batchMethod" cron="0 19 21 * * ?" /> <!--클래스,메서드 및 시간 설정-->
</task:scheduled-tasks>
</beans>
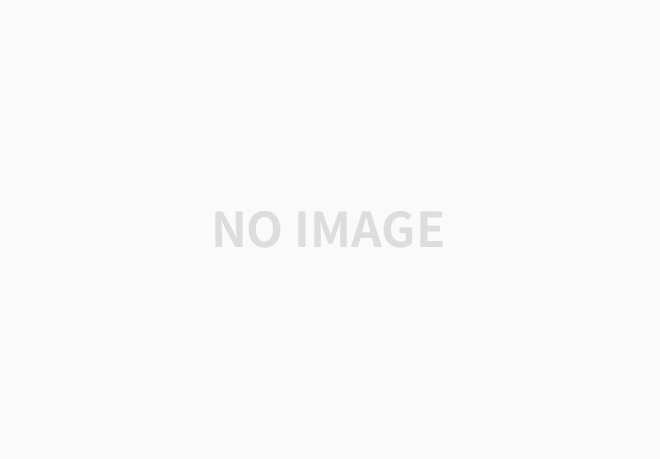
2. service 인터페이스, service구현부는 위와 동일하게 작성하여주면 됩니다.
방법3) @Scheduled 어노테이션 사용
1. 스케줄링 관련 설정을 작성해줍니다.
[context-scheduler.xml]
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-4.0.xsd">
<!-- 컴포넌트 스캔 -->
<context:component-scan base-package="com">
</context:component-scan>
<!-- Annotation 활성화, 컴포넌트스캔과 같이 명시해야합니다. -->
<task:annotation-driven />
<!--배치클래스의 경로-->
<bean id="testJob" class="com.service.serviceImpl.batchServiceImpl" />
</beans>
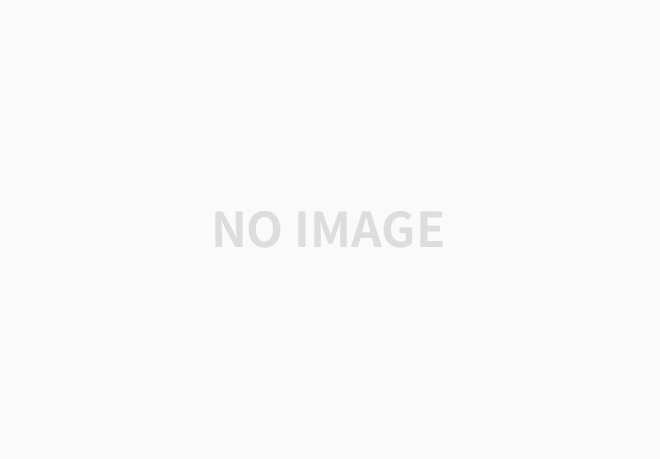
2. service 구현부에 아래와 같이 추가하여줍니다.
package com.service.serviceImpl;
import java.util.HashMap;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
@Service("batchService")
public class batchServiceImpl {
//배치스케줄러 test용
@Scheduled(cron="0 52 21 * * ?")
public void batchMethod() {
HashMap<String, Object> newParam = new HashMap<String, Object>();
System.out.println("배치스케줄러가 정상적으로 동작합니다.");
}
}
@Scheduled(cron="0 52 21 * * ?") 에 동작할 시간형식을 작성하여줍니다.
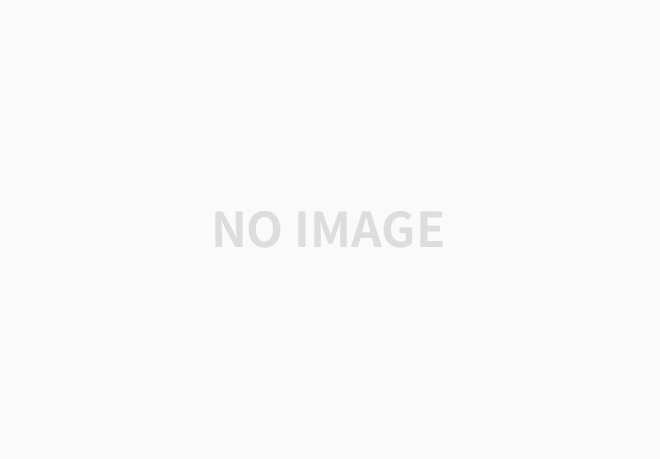
결과
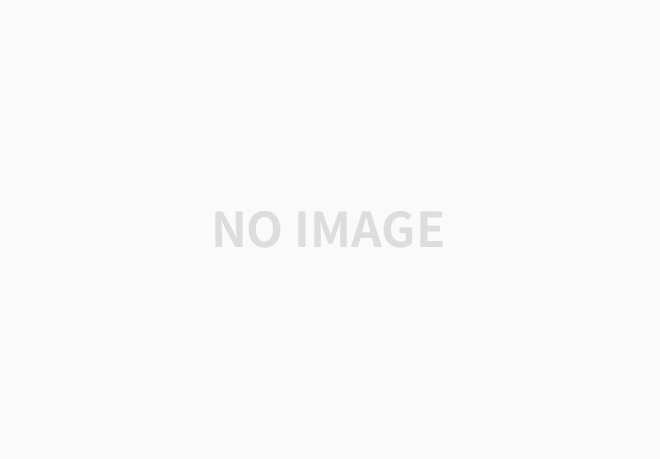
'[Personal_Project]' 카테고리의 다른 글
[Personal_Project] 파일업로드 (단일/다중) (8) (0) | 2021.05.12 |
---|---|
[Personal_Project] DI (필드, 수정자, 생성자)주입방법 (7) (0) | 2021.05.03 |
[Personal_Project] 스프링 시큐리티 기초 (5) (0) | 2021.04.23 |
[Personal_Project] mvc패턴 적용(Dispatcher-servlet) (4) (0) | 2021.04.18 |
[Personal_Project] 이클립스 깃 연동_프로젝트 다운로드 (3) (0) | 2021.04.17 |